What is Git and how do I use it?
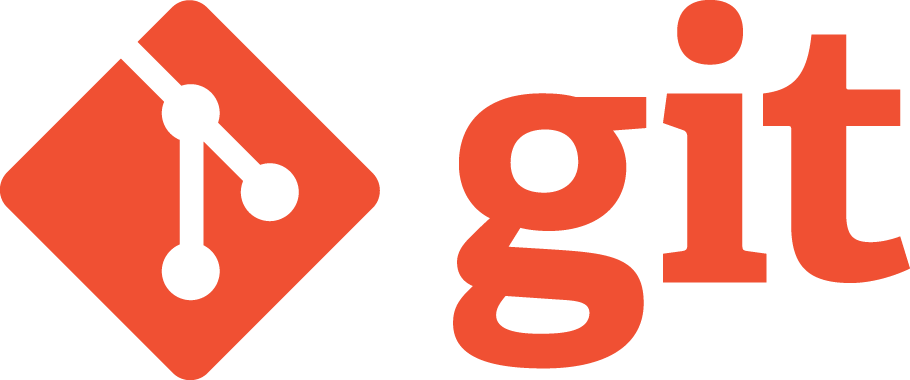
Git is a Version Control System (VCS). By using Git it is possible to share your code with your teammates or other people. In professional software development, it is common sense to use a VCS like Git. When you develop software on your computer and you want to save your changes, you push them to a server. Your teammates can then pull your changes. If they pull your changes they have them on their computers and can continue working on their stuff with your changes already in their code.
How can I create an empty Git repository?
In case that you want to create an empty Git repository, you need to type
git init
Now, the repository is created with one branch named “master”. You can now start working by creating a branch to work on.
What is a branch?
In Git you are working on branches. You can create them, switch between them and delete them. The default branch name in Git is master. The repository in which you are working will contain other branches, too. Often you have a branch called development (or develop) and several other branches which can be named after features or after IDs of features etc. There is no specific schema to name branches except the two common branch names development and master.
When you are working on a branch locally and push the first time to the server, then a branch with the same name as you named it locally will be created on the server. This means you have the same branch two times: locally and remotely. When others want to work on the branch you worked on locally, they can simply checkout this branch from the remote server. This will create a branch with the same name and the same content as the branch on the remote server on their machine. Of course, they will only be able to checkout the revision you pushed most recently to the server and not what you might have added locally, meanwhile. In case that you continue working on your local branch and push changes to the server, your teammates have to pull the latest revision to update their local branch with your latest changes.
We will clarify all the used terms, e.g. push, pull, and checkout later on in this article.
What is a protected branch?
A protected branch is a branch to which you cannot push. The development and master branches should be protected branches to avoid inconsistencies which might create merge conflicts. Another reason for using protected branches is that there may be teammates/people who abuse an unprotected development or master branch to push to them without following your process, e.g. code review or checking test coverage before merging.
How can I create a new branch?
In case that you don’t have the desired branch on your machine and assuming you want to create a branch called “featureXYZ”, you can use the branch command to create a new branch:
git branch featureXYZ
To check out this branch, you need to use the checkout command:
git checkout featureXYZ
In case that you want to create a branch and check it out immediately, you can use the checkout command using the -b
flag:
git checkout -b featureXYZ
In both cases, the branch featureXYZ will be created locally but not on the server. It will be created on the server when you are performing your first push to the server.
How can I create a new branch from an already existing remote branch?
Let’s assume that you have a remote branch called “featureA” and you want to have a local branch of it, you can simply use the checkout command without the -b
flag:
git checkout featureA
In case that there is no remote branch called “featureA”, Git will let you know. It may be possible that you have a typo in the branch name or that it really does not exist.
What is a commit?
A commit is a revision (i.e. a status) of your work to which you can jump later on or which you can revert if it broke your system. It gets a unique identifier (a hash), e.g. 59bc108, which you can use to perform the mentioned actions. In case that you want to checkout this specific revision, you could type
git checkout 59bc108
You could also revert the changes which belong to this revision by using this hash.
How can I create a commit?
Let’s assume you are working on something and finished implementing the tests. You may want to have a single commit pointing to the implemented tests. What you have to do now is
git add .
to add all the changed files to the content of the next commit. You could also add the files one by one in case that you don’t want all files to be added for the next commit by typing
git add MemberControllerTest.java MemberServiceTest.java
What you need to do now is to add a commit message for your commit. You can do it by typing
git commit -m "Implemented tests"
Now, you have added the files to the content of the commit with the message “Implemented tests”. What you need to do now is to push these changes to the remote server.
How can I push the status of my local branch to my server?
When you implemented something and want to save your work remotely, you need to push your changes to the server. All you need to do is use the push command to push all your commits which have not been pushed yet to the server:
git push
You may have guessed: You can create multiple commits and push them to the server with one push command.
Let’s say you are working on branch featureB. It may be possible that the remote branch is not existing yet because you created the branch and it is the first push to this branch. In such a case, Git would let you know that it could not find the branch to push to. What you then need to do is:
git push -u origin featureB
How can I pull the status of a branch from the server into my local branch?
When you want to update your local branch with changes which have already been applied to the corresponding remote branch, you need to type
git pull
Note: Pull is shorthand for
git fetch && git merge FETCH_HEAD
The fetch command fetches branches and/or tags from the remote server and the merge command merges the fetched changes into your local branch.
How can I merge the status of another branch into my current branch?
You are always merging into the branch you are currently on. Assuming you just want to merge another local branch (e.g. “anotherFeature”) without getting its latest revision from the server into your current branch (e.g. “currentFeature”), all you have to do is
git merge anotherFeature
How can I merge the status of another remote branch into my current branch?
Let’s say you are working on branch “currentFeature” and want to have the latest development branch in your feature branch. You need to switch to the development branch, pull from the remote server, go back to your feature branch, and merge your local development branch into the feature branch you are currently on:
checkout development
git pull
git checkout currentFeature
git merge development
You can do this with less commands by staying on the feature branch and pulling to your development branch without checking it out:
git fetch origin development:development
git merge development
The first line updates your local development branch with the latest revision of the development branch on the server (origin). The second line merges your local development branch into the branch you are currently working on.
You could also do this in one line:
git pull origin development:development
The difference is that you are using pull instead of fetch and merge. This command will update your local development branch and merge the changes detected on the remote server into the branch you are currently working on.
Note: The syntax here is the following:
git pull origin local_branch:remote_branch
How do I know what I already changed while working on my branch?
Git has the status command which lets you know
- which branch you are currently working on (marked with an asterisk)
- which files have been added
- which files have been deleted
git status
How can I get a list of all branches?
To get a list of all local branches you need to use the branch command:
git branch
In case that you want to list all local and all remote branches, you need to use the branch command with the -a
flag:
git branch -a
How can I fetch all new branches from the remote server?
During working on your branch, your teammates could have created other branches which are not visible on your machine until you use the fetch command to fetch all new branches from the remote server:
git fetch -a
How can I delete a local branch?
In case that you completed a feature and merged it into your development or master branch, you may want to delete your local branch for this feature. You can delete a local branch by using the branch command with the -d
flag:
git branch -d featureX
To delete a local branch, you should have switched to another branch beforehand.
How can I delete a remote branch?
Sometimes, you want to delete a remote branch, e.g. when a feature has been completed and merged into the development or master branch. You possibly would not want to keep all the feature branches on your server. All you need to do to delete a remote branch is use the push command:
git push origin :featureX
After deleting a remote branch, all teammates need to perform
git fetch --all --prune
to get rid of obsolete tracking branches, i.e. tracking branches of the deleted remote branch.
What is a Merge Conflict?
Let’s say you worked on branch featureX locally and a teammate worked on branch featureY locally. Assuming that there is at least one line which has changed on both branches. In case that your teammate already merged his local branch in the remote development branch and you now want to merge latest revision of the remote development branch into your branch featureX: A merge conflict will occur.
The reason is that there is at least one line which has changed during merging featureY into development and during your local work. Git does not know which of the two changes should be applied. Maybe some lines only have to be put down a little bit and both changes should be applied and none deleted. Git cannot know the logic and intention of your code. You now have to resolve the conflict, which means you have to decide which lines to keep and how to order them.
It is useful to merge the latest revision of the target branch into your local branch before merging into the target branch. If you do so, you can be sure that no additional merge conflicts will occur when merging into the target branch.
What is a Pull Request/Merge Request?
When you are working on a code basis in a team, chances are that you will not be able to merge your implementation into the development or master branch directly. Most of the time, a pull request (sometimes also called merge request) has to be created. This means that you are done with your implementation and want your teammates to review it. Reviewing means that they will have a look at your code with the purpose of improving the code quality. They could check whether you coded according to the coding guidelines, used good names, implemented tests and so on.
Recap
You work on branches which need to be created first. They need to be pushed to the remote server to make them accessible to your teammates. When you worked on your stuff and want to have this status on the corresponding branch on the remote server, you need to create a commit with a commit message. Then, you can push your local changes to the remote server. Now, your teammates are able to pull the latest revision of you branch. When you finished working on your branch and don’t need it anymore, you can delete the branch locally (and remotely). You can also merge branches to combine their content. By doing so, merge conflicts can occur. It is up to you to resolve them because Git cannot know what to keep and what to delete.
Git is a mighty tool to work with several people on the same project. Don’t be afraid of using it just because everything sounds complicated in the beginning. You will need some time to get familiar with Git but by using it day to day, you will master using it.
Reference
For full reference see the Git documentation.