What is the JavaScript Spread Operator?
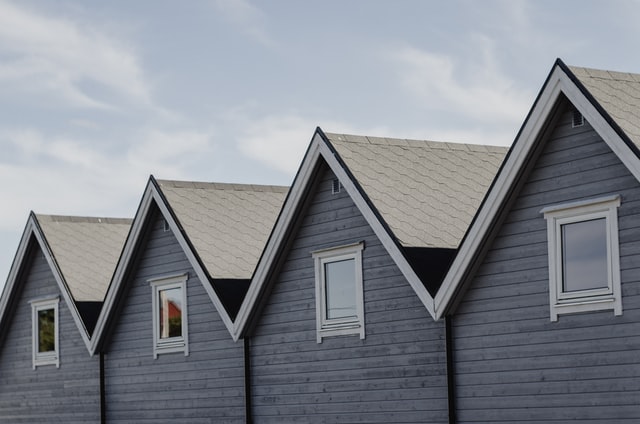
When learning JavaScript, one of the things that I found daunting at first sight was the JavaScript Spread Operator 😱. It looked like magic. I tried to avoid using it for a long time but when I finally tried it out and understood how it worked, I found it pretty useful. In this article, I want to explain what the JavaScript Spread Operator is and how you can use it.
The JavaScript Spread Operator allows you to create an exact copy of the object or array you are using it on. This means, the resulting object will consist of the exact same fields and values as the object you used the Spread Operator on. These clones can be modified without modifying the original object/array. The JavaScript Spread Operator consists of three dots preceding the object or array surrounded by curly braces for objects (e.g. {...user}
) or square brackets for arrays (e.g. [...users]
).
When to use the JavaScript Spread Operator with objects
The Spread Operator can be used for creating exact copies of objects/arrays. These objects or arrays can be modified afterwards or in-place. Another scenario where the Spread Operator comes in handy is when you have an object/array that you would want to keep as is for later use but you need to call a function on this object/array which would manipulate the object/array itself. You could use the Spread Operator to create a copy and call the manipulating function on this copy.
The JavaScript Spread Operator comes in very handy to create a clone of an object:
const user = { name: 'John', age: 33}
console.log(user) // { name: 'John', age: 33}
const userClone = { ...user }
console.log(userClone) // { name: 'John', age: 33}
userClone.name = 'Jane'
console.log(userClone) // { name: 'Jane', age: 33}
console.log(user) // { name: 'John', age: 33}
You can also create a clone of an object and modify it at the same time:
const user = {name: 'John', age: 33}
const modifiedUserClone = { ...user, age: 44}
console.log(modifiedUserClone) // { name: 'John', age: 44 }
The Spread Operator gives you the possibility to create a clone of an object, to modify it and to add additional properties, too:
const user = {name: 'John', age: 33}
const modifiedUserClone = { ...user, age: 34, city: 'Berlin'}
console.log(modifiedUserClone) // { name: 'John', age: 34, city: 'Berlin' }
When to use the JavaScript Spread Operator with arrays
The JavaScript Spread Operator comes in very handy to create a clone of an array and to modify an array, too:
const users = ['John', 'Jane']
console.log(users) // ['John', 'Jane']
const usersClone = [ ...users ]
console.log(usersClone) // ['John', 'Jane']
usersClone.push('Max')
console.log(usersClone) // ['John', 'Jane', 'Max']
console.log(users) // ['John', 'Jane']
const usersClone2 = [...users, 'Max']
console.log(usersClone2) // ['John', 'Jane', 'Max']
console.log(users) // ['John', 'Jane']
Let's say
- you have an array,
- you would need to reverse the array
- but later on you would need the array in its original order:
To do so without the Spread Operator, you would need to reverse the array, perform your operation and reverse it again:
const users = ['John', 'Jane']
console.log('users before function call', users) // ['John', 'Jane']
someFunction(users.reverse())
console.log('users after function call', users) // ['Jane', 'John']
users.reverse()
console.log('users', users) // ['John', 'Jane']
function someFunction(users) {
console.log('in some function', users) // ['Jane', 'John']
}
Instead, you could use the Spread Operator to save one call to the reverse function:
const users = ['John', 'Jane']
console.log('users before function call', users) // ['John', 'Jane']
someFunction([...users].reverse())
console.log('users after function call', users) // ['John', 'Jane']
function someFunction(users) {
console.log('in some function', users) // ['Jane', 'John']
}
Recap
I hope you got an understand of how the Spread Operator works. It allows you to create an exact copy of an object/array. Additionally, it can be used to create clones of objects/arrays and to modify them without modifying the original object/array.
Try to incorporate the Spread Operator in your daily work and you will never want to be without it again 😉